Note
Go to the end to download the full example code.
Testing Ray AABB intersection#
This example shows how to use Ray AABB intersection in matplotlib
10 import matplotlib.pyplot as plt
11 from mpl_toolkits.mplot3d.art3d import Line3DCollection, Poly3DCollection
12
13 import shamrock
18 def draw_aabb(ax, aabb, color, alpha):
19 """
20 Draw a 3D AABB in matplotlib
21
22 Parameters
23 ----------
24 ax : matplotlib.Axes3D
25 The axis to draw the AABB on
26 aabb : shamrock.math.AABB_f64_3
27 The AABB to draw
28 color : str
29 The color of the AABB
30 alpha : float
31 The transparency of the AABB
32 """
33 xmin, ymin, zmin = aabb.lower()
34 xmax, ymax, zmax = aabb.upper()
35
36 points = [
37 aabb.lower(),
38 (aabb.lower()[0], aabb.lower()[1], aabb.upper()[2]),
39 (aabb.lower()[0], aabb.upper()[1], aabb.lower()[2]),
40 (aabb.lower()[0], aabb.upper()[1], aabb.upper()[2]),
41 (aabb.upper()[0], aabb.lower()[1], aabb.lower()[2]),
42 (aabb.upper()[0], aabb.lower()[1], aabb.upper()[2]),
43 (aabb.upper()[0], aabb.upper()[1], aabb.lower()[2]),
44 aabb.upper(),
45 ]
46
47 faces = [
48 [points[0], points[1], points[3], points[2]],
49 [points[4], points[5], points[7], points[6]],
50 [points[0], points[1], points[5], points[4]],
51 [points[2], points[3], points[7], points[6]],
52 [points[0], points[2], points[6], points[4]],
53 [points[1], points[3], points[7], points[5]],
54 ]
55
56 edges = [
57 [points[0], points[1]],
58 [points[0], points[2]],
59 [points[0], points[4]],
60 [points[1], points[3]],
61 [points[1], points[5]],
62 [points[2], points[3]],
63 [points[2], points[6]],
64 [points[3], points[7]],
65 [points[4], points[5]],
66 [points[4], points[6]],
67 [points[5], points[7]],
68 [points[6], points[7]],
69 ]
70
71 collection = Poly3DCollection(faces, alpha=alpha, color=color)
72 ax.add_collection3d(collection)
73
74 edge_collection = Line3DCollection(edges, color="k", alpha=alpha)
75 ax.add_collection3d(edge_collection)
81 def draw_ray(ax, ray, color):
82 """
83 Draw a 3D Ray in matplotlib
84
85 Parameters
86 ----------
87 ax : matplotlib.Axes3D
88 The axis to draw the Ray on
89 ray : shamrock.Ray_f64_3
90 The Ray to draw
91 color : str
92 The color of the Ray
93 """
94 xmin, ymin, zmin = ray.origin()
95 nx, ny, nz = ray.direction()
96 inx, iny, inz = ray.inv_direction()
97 print(ray.direction(), ray.inv_direction())
98 print(nx * inx, ny * iny, nz * inz)
99
100 ax.plot3D([xmin, nx + xmin], [ymin, ny + ymin], [zmin, nz + zmin], c=color)
105 fig = plt.figure()
106 ax = fig.add_subplot(111, projection="3d")
107
108 aabb1 = shamrock.math.AABB_f64_3((-1.0, -1.0, -1.0), (1.0, 1.0, 1.0))
109
110 draw_aabb(ax, aabb1, "b", 0.1)
111
112
113 def add_ray(ray):
114 cd = aabb1.intersect_ray(ray)
115
116 print(cd)
117
118 if cd:
119 draw_ray(ax, ray, "g")
120 else:
121 draw_ray(ax, ray, "r")
122
123
124 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (2.0, 2.0, 2.0)))
125 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (1.0, 2.0, 2.0)))
126 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.7, 2.0, 2.0)))
127 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.6, 2.0, 2.0)))
128 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.5, 2.0, 2.0)))
129 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.1, 2.0, 2.0)))
130 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.0, 2.0, 2.0)))
131 add_ray(shamrock.math.Ray_f64_3((-2.0, -2.0, -2.0), (0.0, 0.0, 2.0)))
132
133 ax.set_xlabel("X")
134 ax.set_ylabel("Y")
135 ax.set_zlabel("Z")
136
137 ax.set_xlim(-2, 2)
138 ax.set_ylim(-2, 2)
139 ax.set_zlim(-2, 2)
140
141 plt.show()
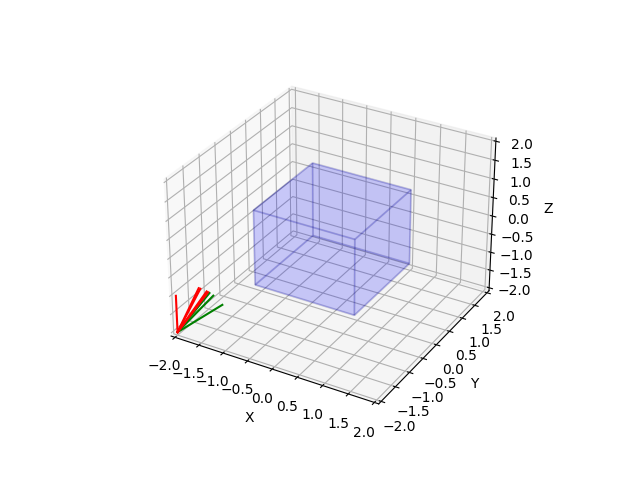
True
(0.5773502691896258, 0.5773502691896258, 0.5773502691896258) (1.7320508075688772, 1.7320508075688772, 1.7320508075688772)
1.0 1.0 1.0
True
(0.3333333333333333, 0.6666666666666666, 0.6666666666666666) (3.0, 1.5, 1.5)
1.0 1.0 1.0
True
(0.24023937806910306, 0.6863982230545802, 0.6863982230545802) (4.1625149383809905, 1.4568802284333466, 1.4568802284333466)
1.0 1.0 1.0
False
(0.20751433915982237, 0.6917144638660746, 0.6917144638660746) (4.8189440982669876, 1.445683229480096, 1.445683229480096)
1.0 1.0 1.0
False
(0.17407765595569785, 0.6963106238227914, 0.6963106238227914) (5.744562646538029, 1.4361406616345072, 1.4361406616345072)
1.0 1.0 1.0
False
(0.03533326266687867, 0.7066652533375732, 0.7066652533375732) (28.30194339616981, 1.4150971698084907, 1.4150971698084907)
1.0 1.0 1.0
False
(0.0, 0.7071067811865475, 0.7071067811865475) (inf, 1.4142135623730951, 1.4142135623730951)
nan 1.0 1.0
False
(0.0, 0.0, 1.0) (inf, inf, 1.0)
nan nan 1.0
Total running time of the script: (0 minutes 0.405 seconds)
Estimated memory usage: 63 MB