Note
Go to the end to download the full example code.
Testing AABB intersection routine#
This example shows how to use AABB intersection and plot it in matplotlib
10 import matplotlib.pyplot as plt
11 from mpl_toolkits.mplot3d.art3d import Line3DCollection, Poly3DCollection
12
13 import shamrock
18 def draw_aabb(ax, aabb, color, alpha):
19 """
20 Draw a 3D AABB in matplotlib
21
22 Parameters
23 ----------
24 ax : matplotlib.Axes3D
25 The axis to draw the AABB on
26 aabb : shamrock.math.AABB_f64_3
27 The AABB to draw
28 color : str
29 The color of the AABB
30 alpha : float
31 The transparency of the AABB
32 """
33 xmin, ymin, zmin = aabb.lower()
34 xmax, ymax, zmax = aabb.upper()
35
36 points = [
37 aabb.lower(),
38 (aabb.lower()[0], aabb.lower()[1], aabb.upper()[2]),
39 (aabb.lower()[0], aabb.upper()[1], aabb.lower()[2]),
40 (aabb.lower()[0], aabb.upper()[1], aabb.upper()[2]),
41 (aabb.upper()[0], aabb.lower()[1], aabb.lower()[2]),
42 (aabb.upper()[0], aabb.lower()[1], aabb.upper()[2]),
43 (aabb.upper()[0], aabb.upper()[1], aabb.lower()[2]),
44 aabb.upper(),
45 ]
46
47 faces = [
48 [points[0], points[1], points[3], points[2]],
49 [points[4], points[5], points[7], points[6]],
50 [points[0], points[1], points[5], points[4]],
51 [points[2], points[3], points[7], points[6]],
52 [points[0], points[2], points[6], points[4]],
53 [points[1], points[3], points[7], points[5]],
54 ]
55
56 edges = [
57 [points[0], points[1]],
58 [points[0], points[2]],
59 [points[0], points[4]],
60 [points[1], points[3]],
61 [points[1], points[5]],
62 [points[2], points[3]],
63 [points[2], points[6]],
64 [points[3], points[7]],
65 [points[4], points[5]],
66 [points[4], points[6]],
67 [points[5], points[7]],
68 [points[6], points[7]],
69 ]
70
71 collection = Poly3DCollection(faces, alpha=alpha, color=color)
72 ax.add_collection3d(collection)
73
74 edge_collection = Line3DCollection(edges, color="k", alpha=alpha)
75 ax.add_collection3d(edge_collection)
80 fig = plt.figure()
81 ax = fig.add_subplot(111, projection="3d")
82
83 aabb1 = shamrock.math.AABB_f64_3((-1.0, -1.0, -1.0), (2.0, 2.0, 2.0))
84 aabb2 = shamrock.math.AABB_f64_3((-2.0, -2.0, -2.0), (1.0, 1.0, 1.0))
85
86 draw_aabb(ax, aabb1, "b", 0.1)
87 draw_aabb(ax, aabb2, "r", 0.1)
88 draw_aabb(ax, aabb1.get_intersect(aabb2), "g", 0.5)
89
90 ax.set_xlabel("X")
91 ax.set_ylabel("Y")
92 ax.set_zlabel("Z")
93
94 ax.set_xlim(-2, 2)
95 ax.set_ylim(-2, 2)
96 ax.set_zlim(-2, 2)
97
98 plt.show()
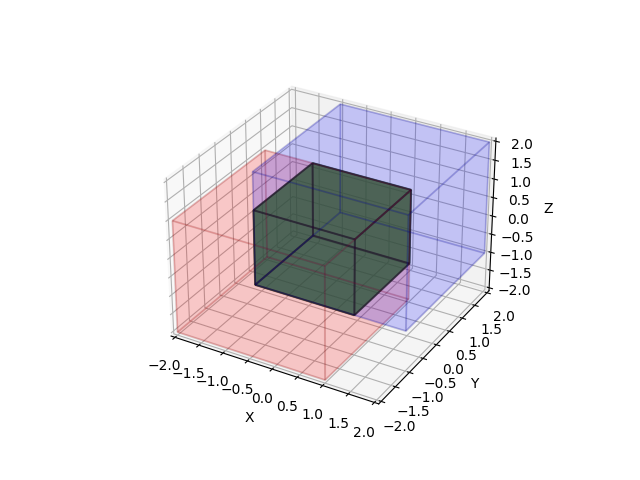
Total running time of the script: (0 minutes 0.368 seconds)
Estimated memory usage: 71 MB